This post will walk through setting up a continuous integration, deployment, and testing pipeline for a Node.js app using CircleCI, Heroku, and Assertible.
Continuous integration is large part of modern development workflows. Most of us are familiar with basic CI services that automatically build code as new changes are pushed. But what if you took that a step further and not only built your code, but also deployed and tested it within the same pipeline? This is continuous development.

Let's outline the steps of the pipeline:
- Continuous integration: Push new code to GitHub, which is then built by CircleCI.
- Continuous deployment: Automatically deploy the new application to Heroku.
- Continuous testing: Execute post deploy smoke tests with Assertible.
I've created an example Node.js app for the code in this post. In the end, you'll have a workflow that will automatically build, deploy, and test your app after every push.
Continuous integration with CircleCI
The pipeline is initiated when code is pushed to a repository on GitHub. CircleCI will automatically pull the new changes, build the code, and run the unit tests. Setting this up only takes two steps:
- Add a
circle.yml
to our repository. You can see the example app's config file in thenodejs-example
repo on GitHub. - Tell CircleCI to watch the project. This is done in your CircleCI dashboard, by adding projects
The .circle.yml
file contains the commands used to build the code.
Since the example app is written in Node.js,
the default settings
will work (npm install
) and you don't need to do anything else.
Now, every time code is pushed to the GitHub repository, CircleCI will run the unit tests and fail if there are any issues:
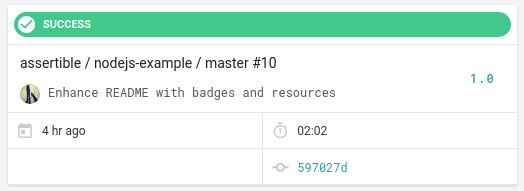
Continuous deployment with Heroku
After our code has been built and the unit tests have passed, CircleCI
will look for a deployment
step in our configuration. In the example
app, I'm deploying the application to Heroku.
In the example project's circle.yml
file, take a look at the
deployment
step again:
deployment:
production:
branch: master
commands:
# ...removed lines for brevity
# Deploy the app to heroku
- git push git@heroku.com:assertible-nodejs-example.git $CIRCLE_SHA1:refs/heads/master
This configuration tells CircleCI to deploy our application to
production when you push to the master
branch of the repo. The
deployment is executed by running each step in commands
.
This is continuous deployment: every time changes are pushed to the repo, CircleCI will build the app, and the deploy it to Heroku. Automatically. Every time. That's pretty cool!
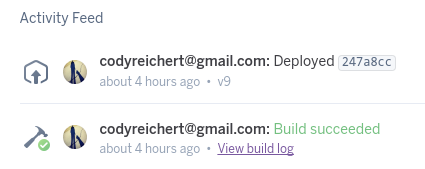
For more on deploying to Heroku, check out the Continuous deployment with Heroku docs on CircleCI or Continuously testing Heroku GitHub deployments blog on Assertible.
Continuous testing with Assertible
After the application is deployed, you want to run automated integration tests on the new version to verify the release and test for critical API failures. To do this, you use Assertible.
Let's look at the last couple of lines in our circle.yml
after
deploying to Heroku:
curl -u $ASSERTIBLE_TOKEN: -XPOST --fail https://assertible.com/deployments -d'{
"service": "'"${ASSERTIBLE_SERVICE_ID}"'",
"environment": "production",
"version": "'"${CIRCLE_SHA1}"'",
"ref": "'"${CIRCLE_SHA1}"'",
"github": true
}'
This code makes request to the Assertible deployments API. When you send a deployment event to Assertible, your API tests are run against the live app. This is post-deployment testing, a part of automated QA testing.
In the test result below, you can see that Assertible ran tests and assertions against the new deployment. It even says what commit the deployment was from:
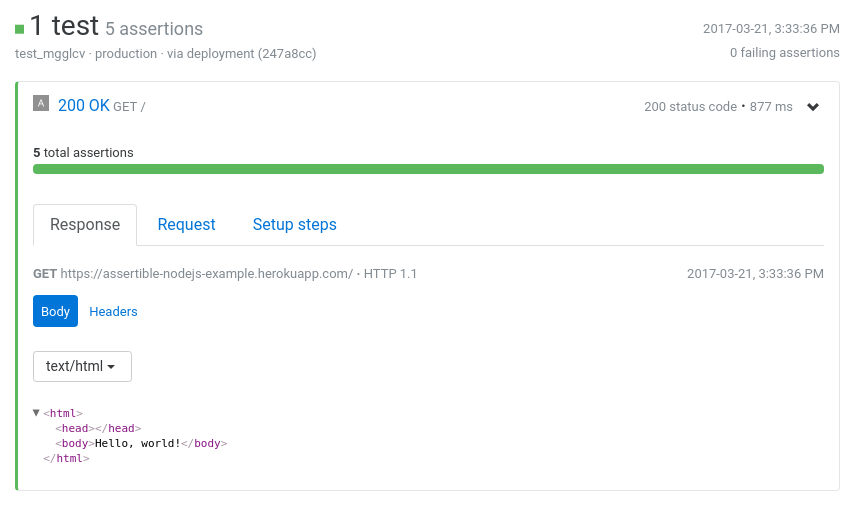
Connecting Assertible to GitHub is easy! Just follow our GitHub Quick Start Guide.
Continuous testing is important for any team or developer releasing software. Automating API and QA tests not only saves time, but covers bugs and issues faster than traditional, manual testing methods.
Continuous testing is important for any team or developer releasing software. Automating API and QA tests saves teams time by identifying broken deployments without manual intervention and provides a baseline quality for your application.
Start building your CI pipeline
Assertible is free to use. Contact us if you have any questions or
feedback!
Testing staging environments from Pull Requests
A lot of times you're not deploying to production, but rather to a staging environment or Heroku review app. Assertible knows about your different environments and will run your API tests on the correct URL.
When you deploy to a staging environment from a GitHub pull request, Assertible will show a status check for API test results that ensures all of your API tests are passing before the new changes are merged. Awesome!
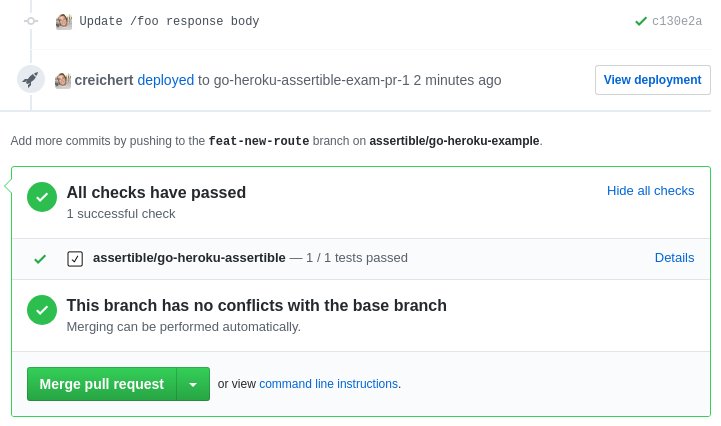
Resources and examples
More automation and integrations
There's even more you can automate, check out some of these resources:
- Heroku has a ton of addons you can use to automate various parts of your build.
- GitHub has a directory of high-quality integrations for your development workflow.
- Assertible has integrations like Zapier and Slack to customize how you run tests and get failure alerts.
Have something to add? Let me know on Twitter!
Integration with other CI services
Nothing about this pipeline is specific to GitHub, CircleCI, Heroku, or even Assertible. Learn more about setting up this pipeline with different CI providers, like Wercker, in the assertible/deployments repo.
What does your continuous development workflow look like? Shoot us a message on Twitter and let us know! Check it out and sign up for a free Assertible account to start testing every single deployment!
Assuredly yours,
Cody Reichert
Categories
The easiest way to test and
monitor your web services
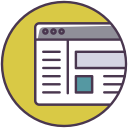
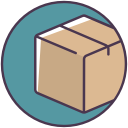
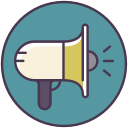
Reduce bugs in web applications by using Assertible to create an automated QA pipeline that helps you catch failures & ship code faster.
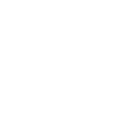
Recent posts
Tips for importing and testing your API spec with Assertible 05/26/2020
New feature: Encrypted variables 10/30/2019
New feature: Smarter notifications 5/17/2019